An In-Depth Analysis of Java's Garbage Collection
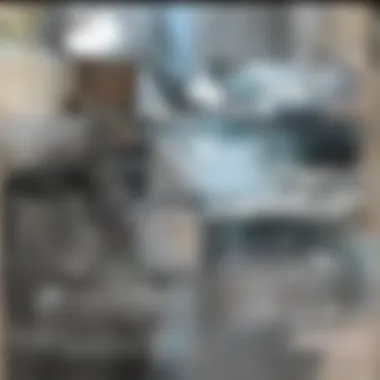
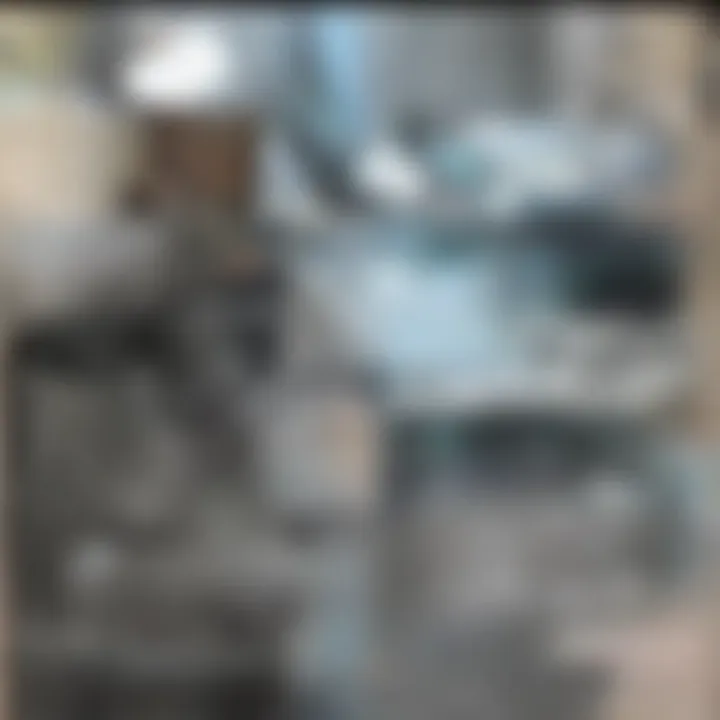
Intro
Java's garbage collection (GC) mechanism is fundamental to memory management within Java applications. Understanding this system is essential for developers, researchers, and educators, as it significantly affects application performance and resource usage. In the journey of software development, managing memory effectively can distinguish between an efficient program and one riddled with memory leaks and sluggishness.
The primary objective of this article is to provide a comprehensive exploration of Java's GC. It will cover the various algorithms implemented, the types of collectors in the Java Virtual Machine (JVM), and the performance implications of GC processes. By recognizing the operational intricacies of GC, the audience can better appreciate its impact on their applications.
Understanding GC can improve the overall experience for developers. With enhanced knowledge, they can adopt better practices for memory management and optimize resource allocation. This article aims to serve as a resource for students, professionals, and researchers who seek a deeper understanding of garbage collection in Java.
Intro to Garbage Collection in Java
Garbage collection, or GC, is vital for memory management in Java. The language automates memory allocation and deallocation, reducing the burden on developers. This section highlights the importance of understanding GC mechanisms, its impact on application performance, and its role in efficient memory management.
In Java, memory handling is not left to the programmer, unlike in languages like C or C++. This automation not only increases productivity but also minimizes memory leaks, a common problem in manual memory management. By utilizing GC, Java helps ensure that unused objects are automatically removed, freeing up resources for new processes.
Understanding how garbage collection works is essential for optimizing applications. It involves recognizing the nuances of different garbage collectors and parameters that can enhance performance. Developers can fine-tune their applications, ensuring they run efficiently without unnecessary memory consumption.
This article will detail the history, fundamentals, algorithms, and best practices related to garbage collection. Through this lens, readers will appreciate the blueprints of Javaβs memory management framework.
What is Garbage Collection?
Garbage Collection refers to the process of automatically identifying and reclaiming memory that is no longer in use by the program. In Java, this is part of the shared responsibility among the Java runtime environment. The basic idea is to remove objects that are not referenced and thus cannot be accessed anymore.
The main intent is to optimize memory usage effectively, thereby preventing memory leaks where unused memory continues to occupy resources. Once the garbage collector identifies that an object is unreachable, it triggers a mechanism to free up that memory for future objects. This process promotes better performance of applications as it allows the system to manage memory dynamically.
Benefits of Garbage Collection
- Automatic Memory Management: Saves programmers from manually deallocating memory.
- Elimination of Memory Leaks: Regular cleaning of unwanted objects helps in maintaining performance efficiency.
- Enhanced Application Performance: By maintaining memory efficiently, the GC can reduce application stalls and enhance user experience.
History and Evolution of Garbage Collection
Garbage Collection in Java has evolved considerably since its inception. Initially, Java utilized a simple mark-and-sweep approach. This method was simplistic but effective for small applications. However, as applications grew in complexity, so did the need for more sophisticated and efficient garbage collections strategies.
Chronology of Key Developments:
- Early 1990s: Java introduced its first garbage collector, mainly mark-and-sweep.
- Late 1990s: Introduction of generational garbage collection, which treats objects differently based on their lifespan.
- 2005 Onwards: The G1 garbage collector was introduced to provide better predictability regarding pause times.
The progress continued with the introduction of modern collectors like the Z Garbage Collector. These advancements greatly improved the scalability of applications, addressing growing demands for performance. The journey from basic techniques to advanced algorithms highlights ongoing growth in handling memory management efficiently.
At the core of Java's trash collection is the fundamental goal of improving memory resource management while reducing developer overhead.
Fundamentals of Memory Management
Memory management is a core concept that underpins the stability and effectiveness of Java applications. Understanding how Java handles memory is essential for developers who wish to optimize their software for performance and reliability. This section explores key components of memory management, including how memory is allocated and deallocated within the Java environment. The focus on memory management is pivotal as it can significantly influence the overall efficiency of Java applications and their responsiveness.
Good memory management leads to better use of resources and can prevent various issues that may arise over time, such as memory leaks and performance degradation. Developers must pay close attention to the management practices to ensure that applications perform optimally under different loads and conditions.
Heap and Stack Memory in Java
Java distinguishes between two primary memory areas: heap memory and stack memory. Understanding these areas is crucial for grasping how Java applications work.
- Heap Memory: This area is used for dynamic memory allocation. Objects created during runtime reside here. The garbage collector regularly scans this memory to reclaim space occupied by objects that are no longer referenced. Heap size can be adjusted to meet the needs of applications, making it flexible but also a potential source of performance issues if not managed carefully.
- Stack Memory: In contrast, stack memory is used for function call management. This area is where method-specific variables are stored. When a method call is completed, its stack frame is removed, and the memory is reclaimed automatically. This makes stack memory very fast but limited in size compared to heap memory.
The different lifecycles and management styles of heap and stack memory should direct how developers allocate memory and foresee potential bottlenecks in performance. By optimizing memory usage in these areas, the application can run more smoothly and efficiently.
Object Allocation and Deallocation
Object allocation and deallocation in Java is fundamentally tied to the garbage collection process. When an object is created, memory is allocated from the heap. The object remains in memory until it is no longer referenced, triggering the garbage collector to reclaim that space. This automatic memory management is one of Java's significant advantages as it frees developers from having to manage memory manually.
The allocation of objects goes hand-in-hand with considerations of how and when those objects are deallocated. Key points to understand include:
- Reference Counting: This method tracks how many references point to an object. When the reference count drops to zero, the object can be safely deallocated.
- Reachability Analysis: The garbage collector employs algorithms to determine which objects are reachable and, by extension, which objects can be cleaned up.
- Finalization: Although not as common in modern Java practices, the finalization method allows for certain cleanup tasks before an object is reclaimed.
By grasping the principles of object allocation and deallocation, developers can create more efficient Java applications that maximize performance and minimize unexpected behavior related to memory handling.
"Efficient memory management in Java ensures an application is not only functional but also scalable and performant, which is critical in todayβs resource-constrained environments."
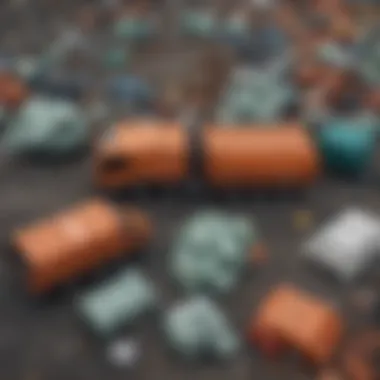
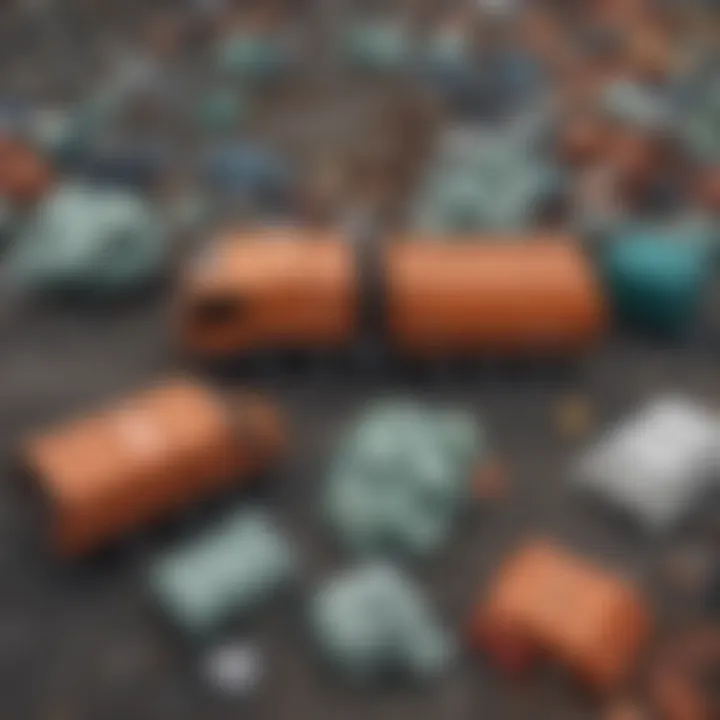
Integrating these concepts into everyday coding practices will aid in mitigating memory-related issues while fostering a deeper understanding of Javaβs memory management strategies.
Types of Garbage Collectors
Understanding the various types of garbage collectors in Java is essential for developers and professionals in the field of software engineering. Garbage collectors play a critical role in managing memory automatically and efficiently. Each type of collector has distinct mechanisms, performance characteristics, and suitable use cases. Choosing the appropriate garbage collector can significantly impact application performance and resource usage.
Serial Garbage Collector
The Serial Garbage Collector is one of the simplest collectors available in Java. It operates in a single-threaded manner, meaning that it pauses all application threads when conducting garbage collection. This approach minimizes the overhead involved in managing multiple threads, making it a good choice for small applications or single-threaded environments. Its primary advantage lies in predictability and ease of implementation.
Despite its benefits, the Serial Collector can introduce latency issues for larger applications when the heap size grows. During garbage collection, the application does not execute, which can lead to noticeable pauses in execution. Therefore, it is usually recommended for applications with small to medium heaps or those requiring minimal pauses.
Parallel Garbage Collector
The Parallel Garbage Collector extends the capabilities of the Serial Collector. It uses multiple threads for minor and major collections, enabling better throughput during garbage collection processes. This design helps utilize multi-core processors efficiently, reducing the impact of garbage collection on application execution time.
A major benefit of the Parallel Garbage Collector is its ability to scale. As the size of the heap increases, the overhead remains manageable due to its concurrent nature. However, applications with extreme real-time requirements may still experience performance hiccups, especially during major collections. Therefore, it is suited for applications that prioritize overall throughput rather than low-latency execution.
Concurrent Mark-Sweep (CMS) Collector
The Concurrent Mark-Sweep (CMS) Collector is designed to minimize pause times caused by garbage collection. It works parallel to application threads, which allows it to reclaim memory without stopping the program for long periods. This behavior makes CMS attractive for applications that require responsiveness.
CMS operates in several phases: initial marking, concurrent marking, concurrent sweeping, and remarking. Each of these phases is designed to execute with minimal interruptions to the application. However, CMS does have some downsides. It can lead to fragmentation of memory, and in some cases, long pauses may occur during the remark phase. Therefore, while it provides better latency performance, careful tuning and monitoring are necessary to ensure optimal results in production environments.
G1 Garbage Collector
The G1 Garbage Collector, or Garbage First Collector, represents a more modern approach to garbage collection in Java. It introduces the concept of regions instead of a monolithic heap. By partitioning the heap into smaller regions, G1 can efficiently manage and prioritize object reclamation based on live data.
G1 aims to provide predictable pause times and operates concurrently with application threads. It includes a root region collection mechanism that minimizes application pause times while maximizing throughput. This collector is particularly well-suited for applications with a large heap and stringent latency requirements. However, being a complex collector, it often requires more tuning and understanding of how it behaves under various loads.
Z Garbage Collector
The Z Garbage Collector (ZGC) is a cutting-edge garbage collector designed for low-latency applications. Support for huge heaps makes ZGC adept at managing very large datasets effectively. It utilizes techniques like concurrent compaction to ensure that the application experiences minimal pause times.
ZGC operates by breaking down the heap into regions and uses a technique called "colored pointers" to track object states, allowing it to relocate objects while the application is running. This collector is especially advantageous for Java applications requiring rapid responses, which is common in cloud and high-performance computing environments. However, ZGC is relatively new, requiring additional understanding and consideration when implementing in production.
Understanding the capabilities and limitations of these garbage collectors allows developers to tailor their memory management strategies effectively.
Garbage Collection Algorithms
Garbage collection algorithms are pivotal in managing Java's memory resources. These algorithms efficiently reclaim memory by identifying and removing objects that are no longer in use. Understanding the varieties and functions of these algorithms is crucial for optimizing application performance. Each algorithm offers unique benefits and trade-offs, depending on the specific needs of the application and the operational context.
The effectiveness of garbage collection algorithms can significantly impact overall application performance, especially in environments with high memory demands. They influence factors such as pause times, throughput, and response times. Therefore, developers must consider the right algorithm for their applications to ensure efficient resource usage.
Mark-and-Sweep Algorithm
The Mark-and-Sweep algorithm is one of the foundational garbage collection strategies used in Java. It functions in two distinct phases: marking and sweeping. In the marking phase, the algorithm begins at the root objects and traverses the object graph, marking all reachable objects. This process ensures that live objects are identified correctly. Once marking is complete, the sweeping phase kicks in. Unmarked objects are deemed unreachable and are subsequently collected, freeing up their allocated memory.
One advantage of this algorithm is its simplicity. It effectively clears memory without the complexity of other methods. However, it can lead to fragmentation, as the sweeping action can leave gaps in memory. As the marked objects leave behind unallocated spaces, performance may degrade over time due to these inefficiencies.
Copying Algorithm
The Copying algorithm takes a different approach compared to the Mark-and-Sweep. This method divides memory into two equal halves. During garbage collection, it only uses one half to allocate new objects, while the other half remains untouched. When collection occurs, the algorithm copies all live objects from the used space into the free space. This not only eliminates unreachable objects but also compacts the surviving objects, resulting in improved memory locality.
The primary benefit of the Copying algorithm is its efficiency. It can avoid fragmentation since all live objects end up in a contiguous block of memory. However, this approach can lead to higher memory usage, as it requires double memory space compared to others. Developers must weigh the trade-offs based on application requirements and memory constraints.
Generational Garbage Collection
Generational Garbage Collection is based on the observation that most objects have a short lifespan, meaning they are quickly created and then discarded. This approach segregates objects into different generations: young, old, and sometimes permanent. The young generation contains newly created objects, while older generations contain objects that have survived several collection cycles.
This algorithm operates mainly on the young generation, typically using a minor collection that is less costly in terms of time. Objects that persist beyond a certain threshold are promoted to the older generation, where they are collected less frequently. This method optimizes collection by focusing on the areas of memory where most garbage is generated, reducing overall collection costs.
"Efficient garbage collection is a cornerstone of performance in modern applications. Developers must understand the options available to optimize memory usage."
For additional information on garbage collection mechanisms, you can refer to Wikipedia).
Performance Considerations
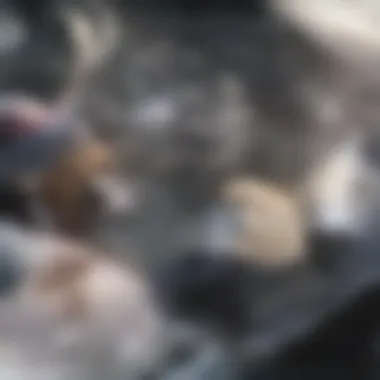
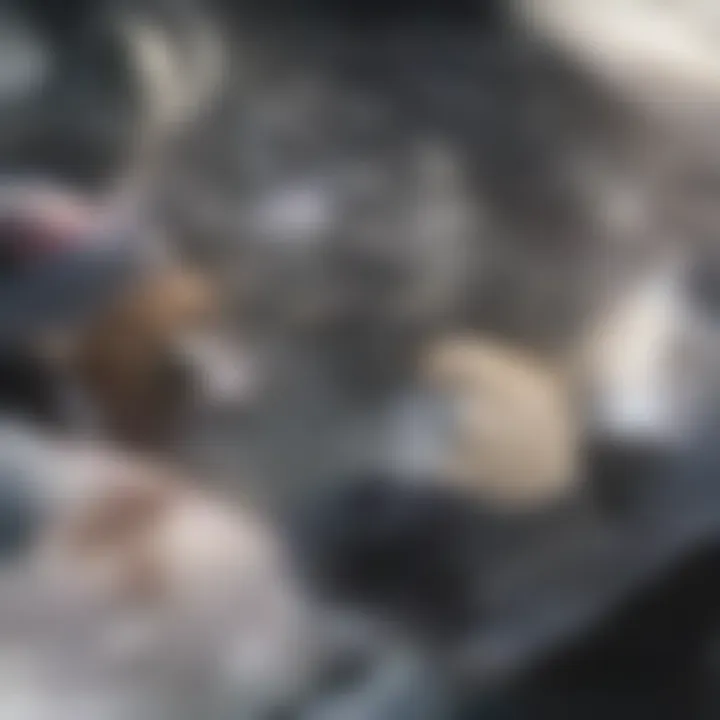
Performance considerations are crucial when discussing Java's garbage collection mechanism. Efficient memory management can significantly affect application responsiveness and resource utilization. Understanding how garbage collection works and its implications on performance allows developers to make informed decisions that enhance their applications' efficiency.
In Java, the performance of an application can deteriorate due to several reasons. One of these is the garbage collection process, which, while necessary for reclaiming memory, can introduce latency. The overall efficiency is often evaluated in terms of pause times, memory footprint, and throughput. Tuning the garbage collector can help mitigate these issues, but it requires an understanding of various factors that influence performance.
Impact on Application Performance
Garbage collection can impact application performance in both positive and negative ways. On the positive side, it helps manage memory automatically. This feature increases developer efficiency, reducing the likelihood of memory leaks or manual deallocations that can lead to crashes. However, there are trade-offs.
The garbage collection process uses CPU cycles, which can lead to performance hits when applications experience sporadic delays, known as GC pauses. These pauses are a direct consequence of the algorithms used by JVM's garbage collectors. High-frequency collection or prolonged pauses can create noticeable latency, especially in real-time applications.
- Major Factors Affecting Performance:
- Selection of the appropriate garbage collector for the applicationβs workload
- Frequency and duration of GC pauses
- Size of the Java heap and memory used by the application
Understanding these factors helps developers optimize the performance of their applications. Choosing the right garbage collector can improve overall throughput by effectively balancing the workload.
Tuning Garbage Collection
Tuning garbage collection involves adjusting the parameters and settings of the garbage collector to improve application performance. This process can be intricate, as it depends on the specific needs of the application and its environment.
Typically, tuning includes:
- Heap Size Configuration: Properly setting initial and maximum heap sizes can reduce the frequency of garbage collection. A larger heap can accommodate more objects, leading to fewer collections, but it can also increase pause times.
- Choosing the Right Collector: Depending on the application type, developers may select a collector that optimizes pause time or throughput. For instance, the G1 collector is suited for applications that require predictable pause times.
- Utilizing JVM Options: Java provides several command-line options for tuning GC behavior. For example, flags like select the respective garbage collector, while can help in managing pause durations.
By implementing these adjustments, developers can greatly enhance application performance.
"Effective tuning of garbage collection can lead to significant improvements in both response time and throughput of Java applications."
Overall, performance considerations are a foundational aspect of Java's garbage collection mechanism. By understanding these factors, developers can create optimized applications that are both efficient and reliable, meeting the demands of users and stakeholders.
Common Challenges in Garbage Collection
Garbage collection in Java is essential for memory management, yet it comes with its share of challenges. Understanding these challenges is crucial for developers seeking to optimize application performance and maintain stability in Java environments.
Two significant issues often arise during garbage collection: memory leaks and out of memory errors. Each of these can affect the efficiency of an application and its overall user experience. Having a detailed knowledge of these problems can help developers devise strategies to prevent them.
Memory Leaks
Memory leaks occur when an application retains references to objects that are no longer needed. This is often due to improper handling of object references or failing to release resources. In Java, the garbage collector is supposed to reclaim memory from objects that are unreachable, but if there are lingering references, the memory occupied by these objects remains allocated.
Common causes of memory leaks include:
- Static Collections: When a static variable holds onto objects, they cannot be garbage collected until the class is unloaded.
- Listeners or Callbacks: If event listeners are not removed, they may keep objects alive beyond their intended lifecycle.
- Caching Mechanisms: Sometimes, objects are cached without appropriate eviction strategies, leading to unintended retention of memory resources.
Developers should implement careful practices to avoid memory leaks. Utilizing tools like profilers can help detect such leaks. Regularly reviewing code and structure can also mitigate risks associated with these leaks.
Out of Memory Errors
Out of memory errors occur when the Java application fails to allocate enough memory for new objects. This situation can arise even with garbage collection active, as the Java Virtual Machine (JVM) may run out of memory pools or face fragmentation issues. These errors directly impact application stability and user experience.
There are several types of out of memory errors:
- Java heap space: This is one of the most common errors, typically indicating that the heap memory cannot accommodate new objects.
- PermGen space: In older Java versions, this error indicates that the permanent generation area, which stores class metadata, is full.
- Native memory: This error arises when the application exhausts the memory allocated from the native memory for JNI calls and other operations.
To address out of memory errors, developers can opt for several measures such as:
- Increasing JVM Memory: Allocating more memory to the JVM can often alleviate these errors, but it is a temporary fix.
- Optimizing Code: Analyzing memory usage patterns and optimizing object creation can reduce the risks of such errors.
- Using Profiling Tools: These tools can help track memory consumption and identify where optimization is needed.
Monitoring and understanding memory usage is vital for maintaining application performance and preventing the risks associated with memory management issues.
In summary, memory leaks and out of memory errors are significant challenges that developers must address to ensure efficient garbage collection in Java. Awareness and proactive measures can facilitate better resource management and improve overall application performance.
Best Practices for Developers
Understanding best practices for developers in the context of Java's garbage collection is vital for optimizing application performance and resource management. Effective garbage collection strategies can significantly reduce memory leaks and application latency, enhancing overall system reliability. This section delves into key considerations and methodologies that developers should adopt to ensure efficient memory usage and effective monitoring mechanisms.
Designing for Efficient Memory Usage
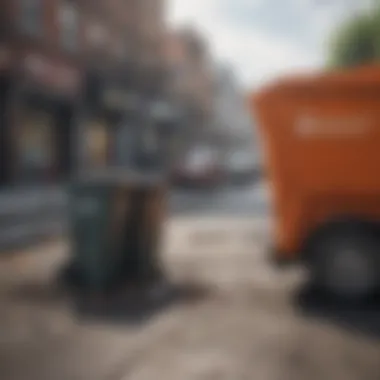
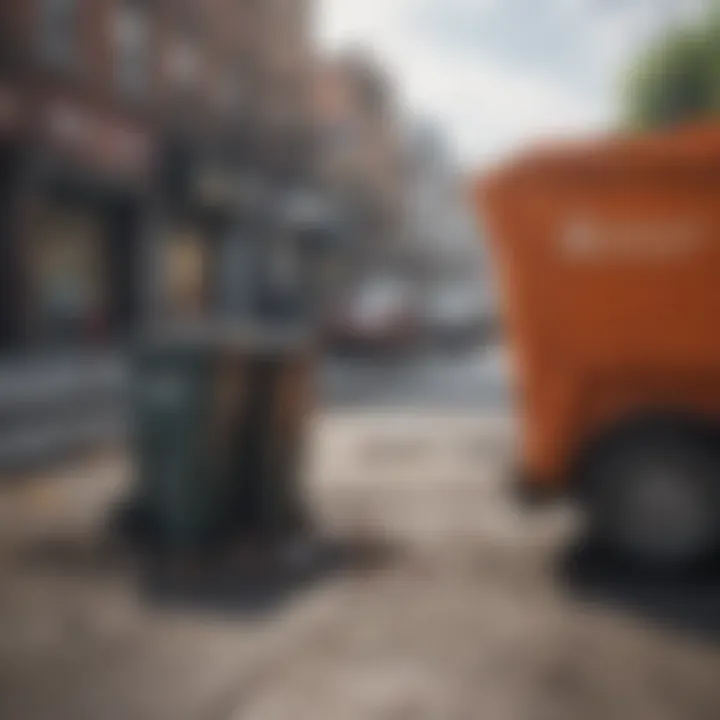
Efficient memory usage starts at the design phase of application development. Developers must consider how objects are created, retained, and disposed of in memory. Here are some effective strategies:
- Limit Object Creation: Excessive instantiation of objects can overwhelm the garbage collector. Using object pooling can mitigate this issue, allowing for reuse of objects instead of constant creation and destruction.
- Choose Appropriate Data Structures: The selection of data structures impacts memory usage. Understanding the trade-offs between options like and or using wisely can optimize memory allocation and retrieval times as well.
- Release References Promptly: Failing to clear references to unused objects can lead to memory leaks. Ensuring local variables go out of scope and nullifying long-lived references when no longer needed can help the garbage collector recover these resources efficiently.
- Avoid Unnecessary Finalizers: Using finalizers can increase the overhead on garbage collection. Instead, consider using for closing resources appropriately, or implement interfaces when applicable.
By implementing these strategies, developers place themselves in a position to reduce workload on the garbage collector, thereby optimizing the efficient allocation of memory.
Monitoring and Profiling Applications
Monitoring and profiling are essential practices that help developers gain insights into the memory usage and performance of their applications. Identifying bottlenecks can lead to more informed decisions about memory management. Here are several techniques:
- Use Profiling Tools: Tools such as VisualVM, JProfiler, and YourKit provide sophisticated metrics on memory usage and heap dumps. These tools can help identify memory leaks and track down inefficient code paths that might cause undesired memory consumption.
- Analyze Garbage Collection Logs: Enabling GC logging offers a window into how often garbage collection occurs, and the duration of collection cycles. Frequent pauses may indicate that the application requires optimization.
- Implement Application Metrics: Leveraging frameworks like Micrometer or Java Management Extensions (JMX) allows developers to create metrics on memory usage that can be monitored in real time. This proactive approach helps in anticipating performance degradation before it impacts users.
By incorporating these monitoring practices, developers can proactively manage application performance and memory usage, facilitating timely interventions when required.
In summary, best practices for efficient memory usage and monitoring are essential to mastering Java's garbage collection mechanism, ultimately leading to a sustainable and high-performing application.
Future Directions of Garbage Collection
The future of garbage collection (GC) is an area of active research and exploration within the realm of Java programming. As applications become increasingly complex and the demand for resource efficiency grows, understanding the upcoming advancements in GC is vital. These developments may greatly enhance application performance and memory management.
Several specific elements come into play when discussing future directions in GC. First, machine learning integration stands out. Leveraging machine learning can offer smarter strategies to determine when and how to collect garbage. Non-trivial patterns in memory usage can be identified. This offers the potential for more adaptive and efficient garbage collection processes.
Another critical consideration is the continual advancement of garbage collection algorithms themselves. Existing algorithms may be optimized further. This could lead to reduced pause times and better overall performance. Understanding these innovations will prepare developers to harness them effectively in their applications.
The exploration of these future directions can bring multiple benefits. Faster and more efficient GC reduces application downtime, which is crucial for real-time systems. More efficient algorithms can lower resource usage, which is especially important in cloud environments.
"As applications scale, optimizing garbage collection through innovative techniques is not just an option; it is a necessity."
In essence, keeping an eye on the future of garbage collection equips developers with the tools and knowledge to build cutting-edge applications. Understanding these trends provides a foresight advantage that can guide design and implementation choices.
Machine Learning in GC
Machine learning holds significant promise for optimizing garbage collection in Java. It can analyze vast amounts of runtime data to identify patterns that indicate when memory usage spikes occur. This allows the system to make better predictions about garbage collection needs.
By implementing algorithms that learn from previous behaviors, automated tuning of garbage collection parameters can become a reality. If the system learns that certain objects tend to be short-lived or long-lived, it can adjust its collection strategy accordingly. This adjustment may lead to fewer interruptions and increased throughput, as the GC can work smarter, not just harder.
Some advantages of machine learning applications in GC include:
- Dynamic adjustment: GC can adjust its behavior in real-time based on learned data.
- Reduced overhead: Less frequent, but more targeted collections can minimize CPU overhead.
- Better resource allocation: Improves how memory resources are allocated and freed.
Research in this area is still evolving. However, early implementations have shown encouraging results in terms of both performance and efficiency.
Advancements in GC Algorithms
As the landscape of programming evolves, so do garbage collection algorithms. One area of focus is refining existing algorithms to strike a balance between performance and resource consumption. For example, integration of more granular tuning options within algorithms enables developers to tailor garbage collection strategies based on specific application needs.
Innovations like predictive algorithms and generational approaches continue to shape future prospects. Predictive algorithms utilize runtime statistics to forecast memory needs. Generational garbage collection takes advantage of the observation that most objects tend to die young. This helps in structurally optimizing the collection process.
To enhance practical performance, developers are actively researching:
- Lower latencies: Reducing pause times continues to be a primary challenge.
- Improved compaction strategies: Efficiently rearranging live objects in memory to reduce fragmentation is key.
- Parallelism: Enhancing the ability to run multiple collection threads can further optimize CPU usage.
Advancements in these areas may lead to the creation of more intelligent garbage collectors. These enhancements promise to deliver greater performance gains and superior application responsiveness.
Culmination
Understanding garbage collection in Java is essential for optimizing application performance and resource management. This article highlights the intricacies of the Java garbage collection mechanism, including its various algorithms, collectors, and performance considerations. Through a comprehensive exploration of these elements, we underline the profound impact garbage collection has on application responsiveness and memory efficiency.
Summary of Insights
The insights presented throughout this article clarify that:
- Garbage Collectors: Different types of garbage collectors, including Serial, Parallel, Concurrent Mark-Sweep, G1, and Z, serve distinct use cases. Choosing the right collector is crucial based on application requirements.
- Algorithms: Understanding algorithms such as Mark-and-Sweep, Copying, and Generational GC can help in selecting the right strategy for memory reclamation.
- Performance: The performance of applications can be significantly influenced by how effectively garbage collection is tuned and monitored.
- Best Practices: Implementing best practices in memory management leads to more efficient applications, minimizing memory leaks and out of memory errors.
Overall, recognizing the role of garbage collection helps in writing cleaner, more efficient code, thus improving overall user experience and resource utilization.
Importance of Understanding GC
Garbage collection is not just a background task but a vital component of Java performance. A profound understanding of this topic enables developers, students, and professionals to create applications that:
- Run efficiently with minimal overhead during memory management processes.
- Avoid common pitfalls such as memory leaks, contributing to more robust applications.
- Make informed decisions about memory allocation and deallocation strategies.
- Utilize profiling and monitoring tools effectively, enhancing application stability.
The importance of comprehending garbage collection cannot be overstated. It is integral for anyone working within the Java ecosystem. Whether be it for academic purposes, research, or practical application development, the ramifications of garbage collection are vast and influence numerous facets of programming in Java.